Swashbuckle and Swagger generates useful documentation for Web APIs. It can also allow you to call the API from your browser.
For example:
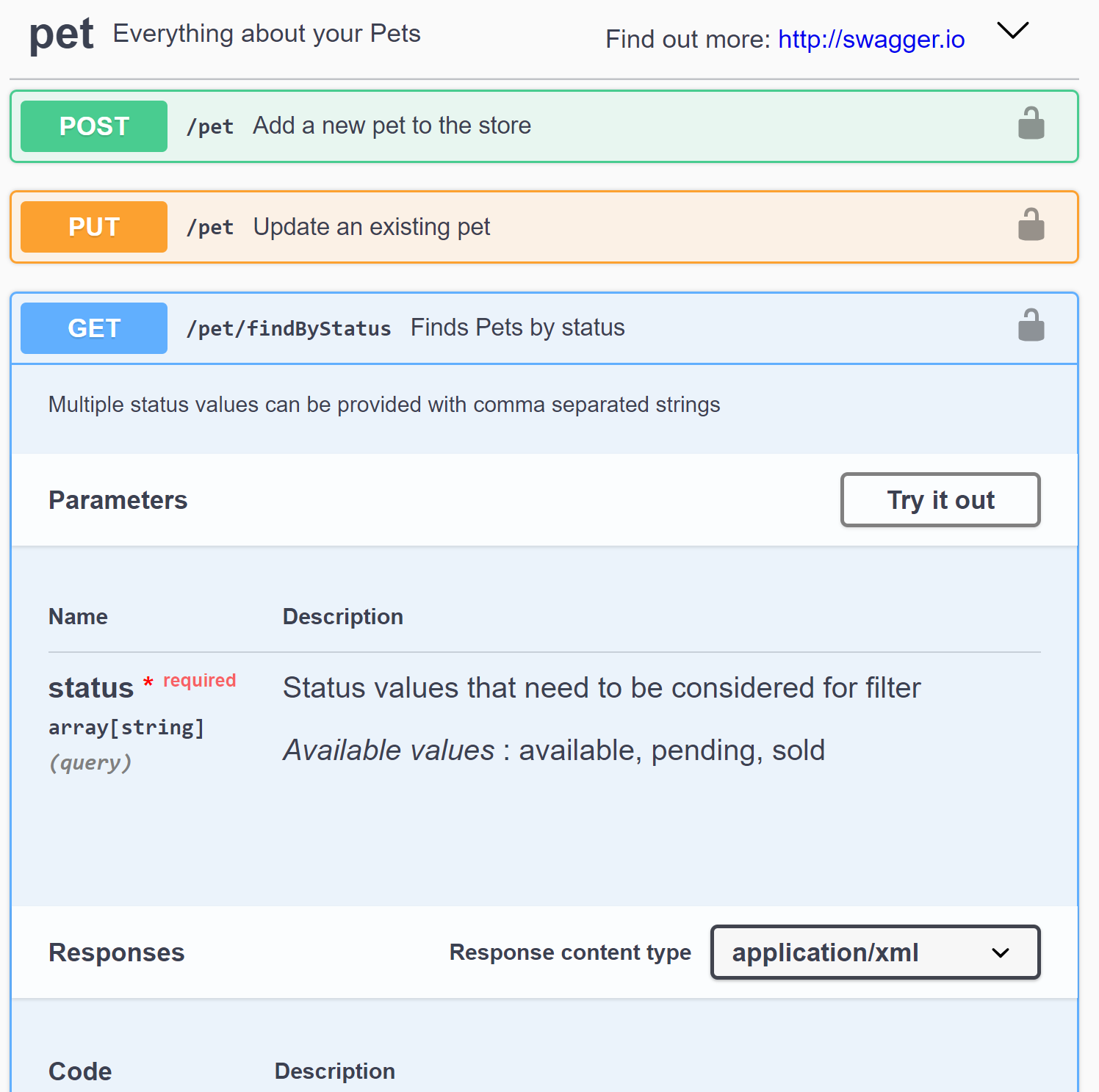
Swashbuckle generates Swagger document objects directly from your routes, controllers, and models. The Swagger documentation is automatically generated using SwaggerUI and is displayed when you browse to your API.
This post gives a quick start guide on getting up and running with Swagger. More information can be found at https://swagger.io and https://github.com/domaindrivendev/Swashbuckle
To setup it up in .Net Core:
Install-Package Swashbuckle.AspNetCore
Statup.cs
In Startup.cs, import the following namespace:
using Swashbuckle.AspNetCore.Swagger;
Add the following to Startup.ConfigureServices:
services.AddSwaggerGen(c =>
{
//Change Title and Version to customize the text used in the Swgger UI
c.SwaggerDoc("v1", new Info { Title = "My API", Version = "v1" });
});
Add the following to Startup.Configure:
// Enable middleware to serve generated Swagger as a JSON endpoint.
app.UseSwagger();
// Enable middleware to serve swagger-ui (HTML, JS, CSS, etc.),
// specifying the Swagger JSON endpoint.
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "My API V1");
c.RoutePrefix = string.Empty;
});
app.UseMvc();
Decorate your API actions:
Let Swagger know what the expected responses types are likely to be, e.g.:
[ProducesResponseType(200)]
[ProducesResponseType(401)]
public IActionResult Index()
{
....
}
Including your own comments
If you want to include comments from your code into the Swagger UI:
Update your project so it generates an XML documentation file:
- Right click project and click properties
- In the Build tab, tick the “XML documentation file” box
Update Start.cs: Update the following in Startup.ConfigureServices:
services.AddSwaggerGen(c =>
{
//Change Title and Version to customize the text used in the Swgger UI
c.SwaggerDoc("v1", new Info { Title = "My API", Version = "v1" });
// Set the comments path for the Swagger JSON and UI.
var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml";
var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile);
c.IncludeXmlComments(xmlPath);
});
Add comments to your API action. These will be picked up by Swashbukle and displayed in your Swagger UI.
/// <summary>
/// Query the job status
/// </summary>
/// <remarks>
///
/// </remarks>
/// <param name=”id”>The ID of the job to check</param>
/// <response code=”200″>Returns the status of the job</response>
/// <response code=”404″>The job with the requested ID could not be found</response>
/// <response code=”500″>Server error</response>
public IActionResult Get(int id)
{
....
}